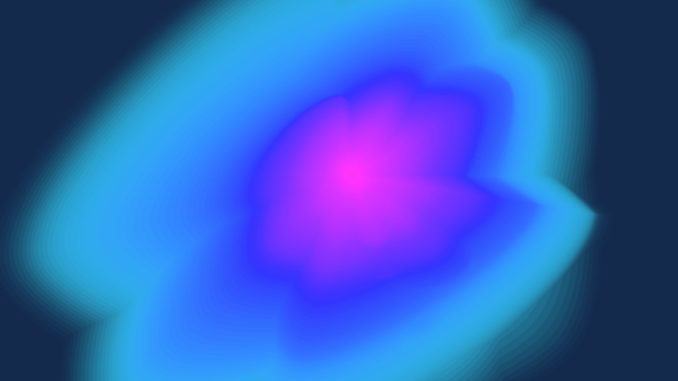
Cette expérimentation consiste en l’évolution d’une forme complexe et mouvante, en fonction de la valeur d’un capteur Arduino(potentiomètre, capteur lumineux,…).
Pour cela, nous avons dû passer par p5js, plus performant que l’écriture Java.
afin de connecter la carte arduino a p5js, nous somme passés par le site https://editor.p5js.org/ et avons téléchargés le logiciel p5 serial control.
Code arduino: lire valeur capteur arduino
const int potPin = A0; // pin the pot is connected to
int potVal = 0; // variable to hold the value from the sensor
void setup() {
// initialize the serial communication:
Serial.begin(9600);
}
void loop() {
potVal = analogRead(potPin);
// read the value of the pot and store it
potVal=map(potVal,0,1024,20,200);
// write the value out to the serial port
Serial.println(potVal);
// wait a bit for between readings
delay(100);
}
Code HTML :
<html lang="en">
<head>
<script src="https://cdnjs.cloudflare.com/ajax/libs/p5.js/0.10.2/p5.js"></script>
<script language="javascript"type="text/javascript"src="https://cdn.jsdelivr.net/npm/p5.serialserver@0.0.28/lib/p5.serialport.js"></script>
</head>
<body>
<script src="sketch.js"></script>
</body>
</html>
Code CSS :
html,
body {
margin: 0;
padding: 0;
}
canvas {
display: block;
}
Code p5.js :
let serial; // variable for the serial object
let latestData = "waiting for data"; // variable to hold the data
let kMax; // maximal value for the parameter "k" of the blobs
let step = 0.01; // difference in time between two consecutive blobs
let n = 100; // total number of blobs
let radius = 0; // radius of the base circle
let inter = 0.05; // difference of base radii of two consecutive blobs
let maxNoise = 500;
function setup() {
createCanvas(windowWidth, windowHeight);
// serial constructor
serial = new p5.SerialPort();
// serial port to use - you'll need to change this
serial.open("COM6");
// what to do when we get serial data
serial.on('data', gotData);
colorMode(HSB, 1);
noFill();
//noLoop();
kMax = random(0.6, 1.0);
noStroke();
}
// when data is received in the serial buffer
function gotData() {
let currentString = serial.readLine(); // store the data in a variable
trim(currentString); // get rid of whitespace
if (!currentString) return; // if there's nothing in there, ignore it
console.log(currentString); // print it out
latestData = currentString; // save it to the global variable
}
function draw() {
text(latestData, 10, 10); // print the data to the sketch
background(0.6, 0.75, 0.30);
let t = frameCount/(latestData*1.25);
for (let i = n; i > 0; i--) {
let alpha = 1 - (i / n);
fill((alpha/(latestData/15) + 0.5)%1, 0.8, 1, alpha);
let size = radius + i * inter;
let k = kMax * sqrt(i/n);
let noisiness = maxNoise * (i / n);
blob(size, width/2, height/2, k, t - i * step, noisiness);
}
}
function
blob(size, xCenter, yCenter, k, t, noisiness) {
beginShape();
let angleStep = 360 / 10;
for (let theta = 0; theta <= 360 + 2 * angleStep; theta += angleStep) {
let r1, r2;
/*
if (theta < PI / 2) {
r1 = cos(theta);
r2 = 1;
} else if (theta < PI) {
r1 = 0;
r2 = sin(theta);
} else if (theta < 3 * PI / 2) {
r1 = sin(theta);
r2 = 0;
} else {
r1 = 1;
r2 = cos(theta);
}
*/
r1 = cos(theta)+1;
r2 = sin(theta)+1; // +1 because it has to be positive for the function noise
let r = size + noise(k * r1, k * r2, t) * noisiness;
let x = xCenter + r * cos(theta);
let y = yCenter + r * sin(theta);
curveVertex(x, y);
}
endShape();
}
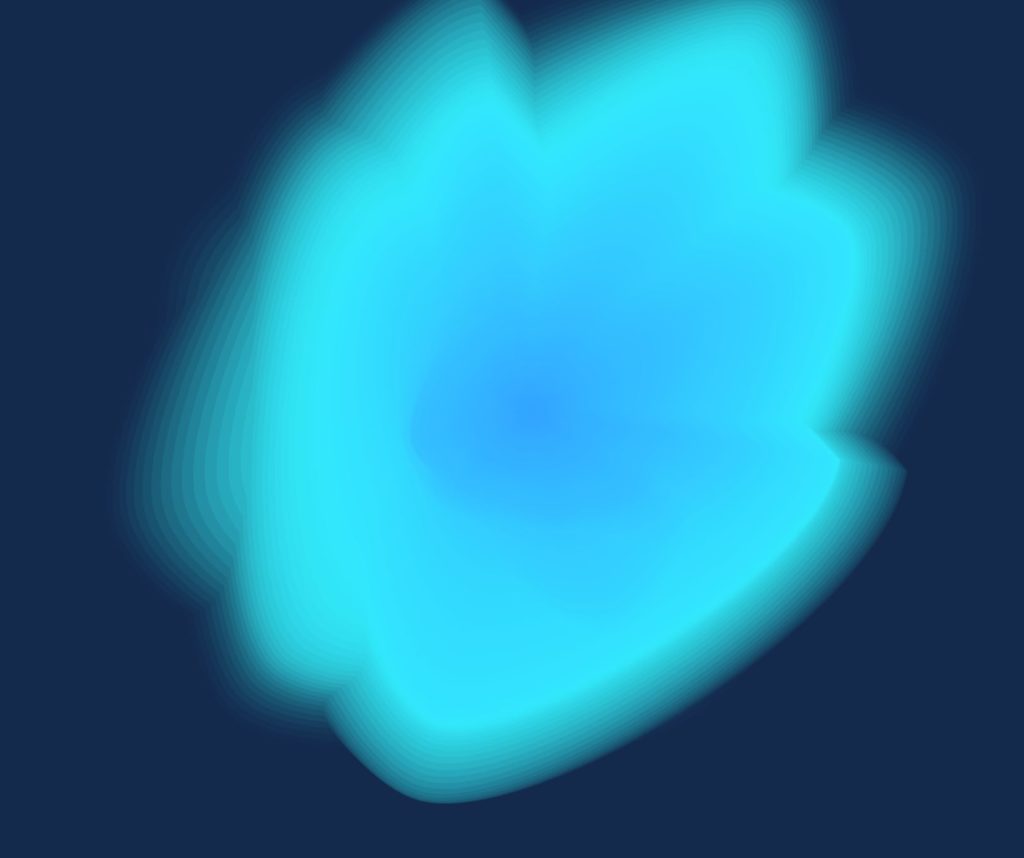
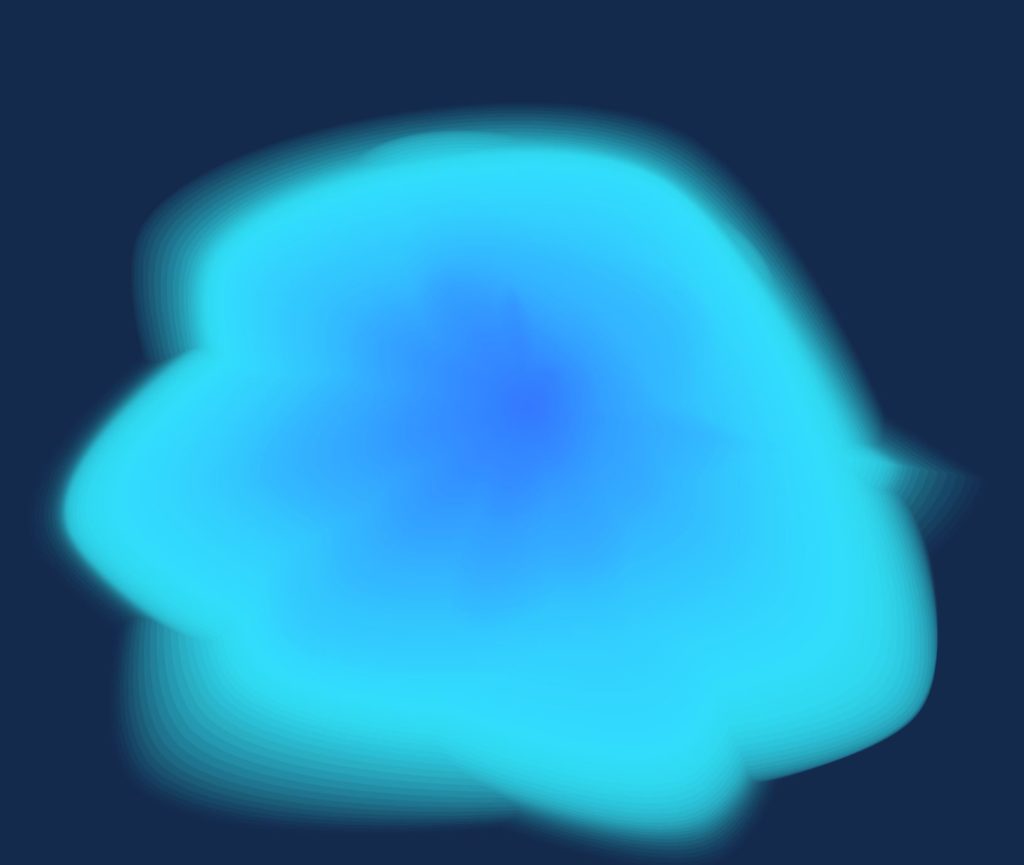
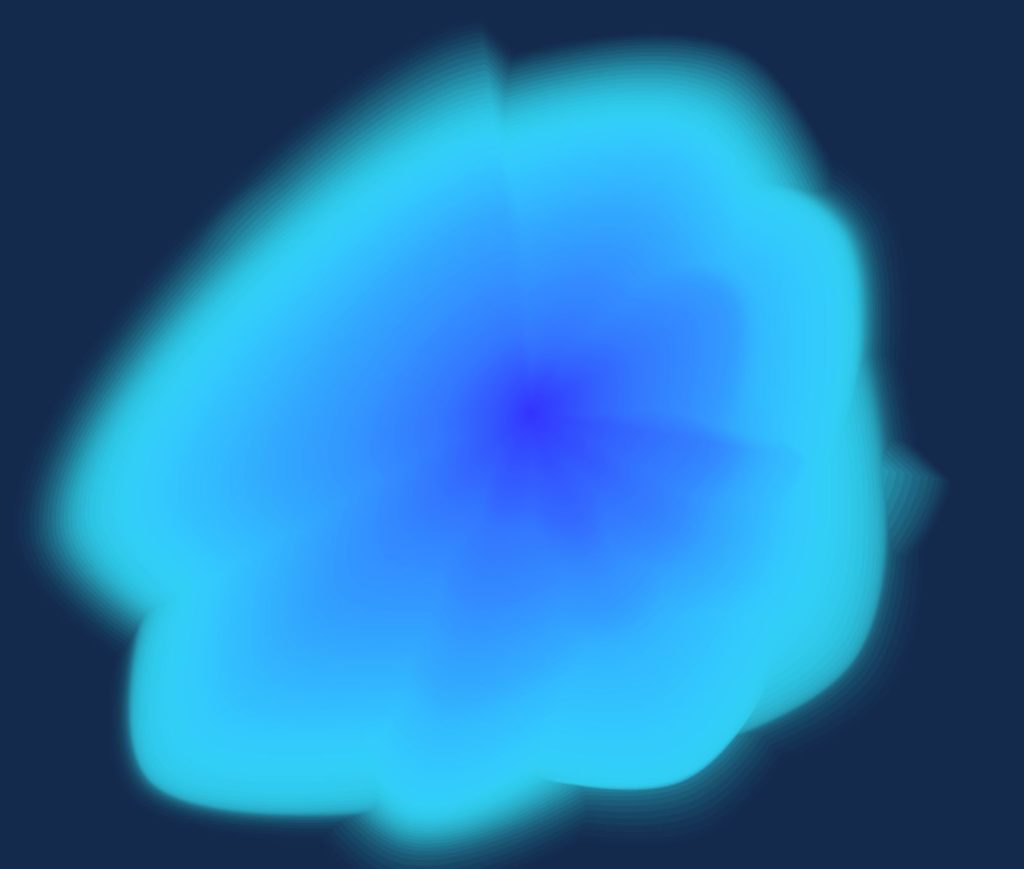
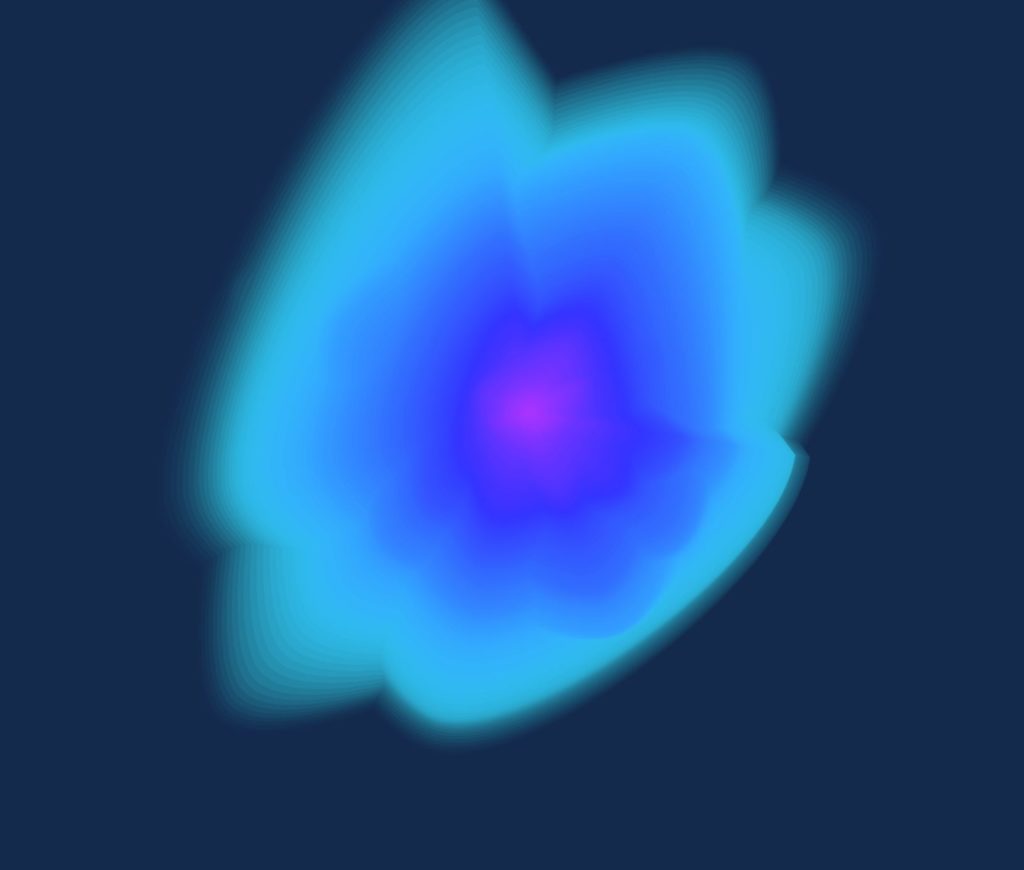
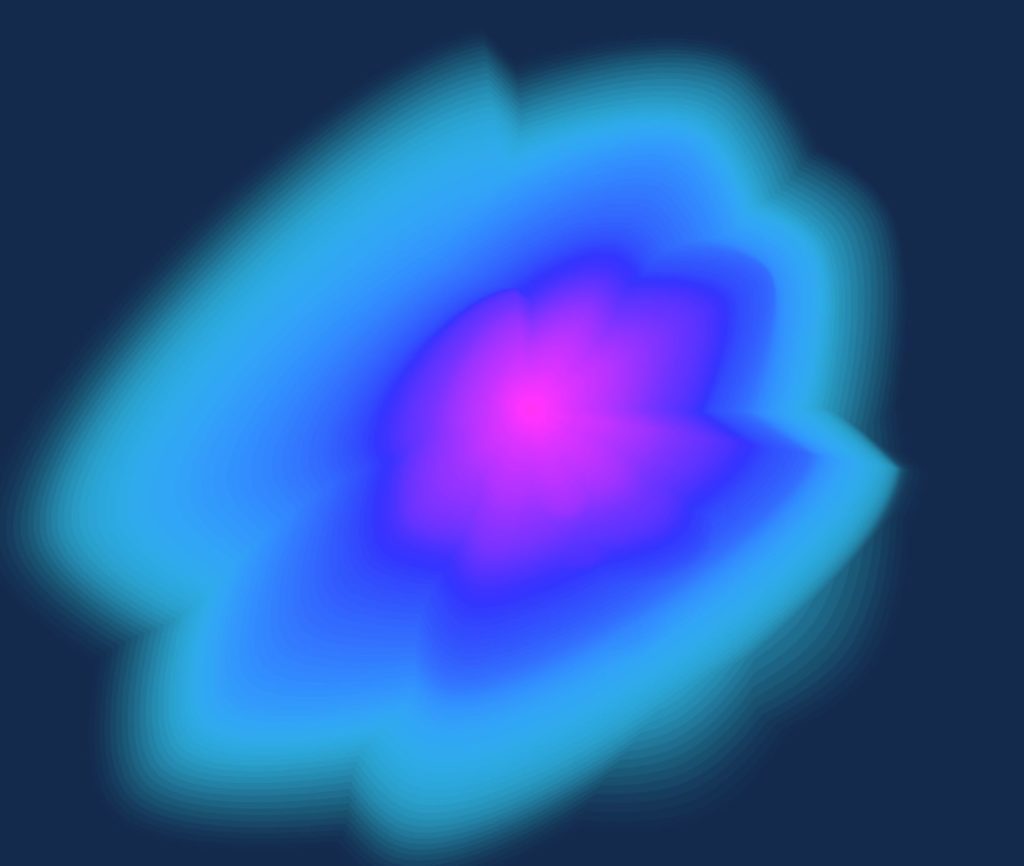
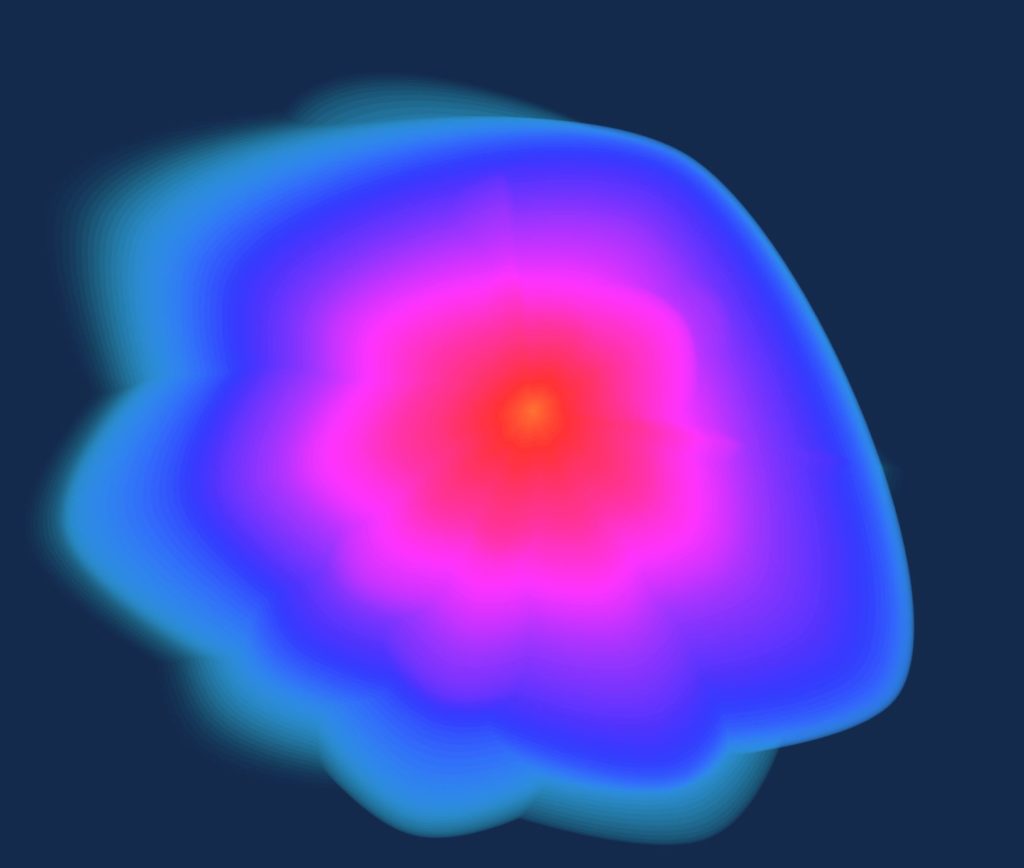
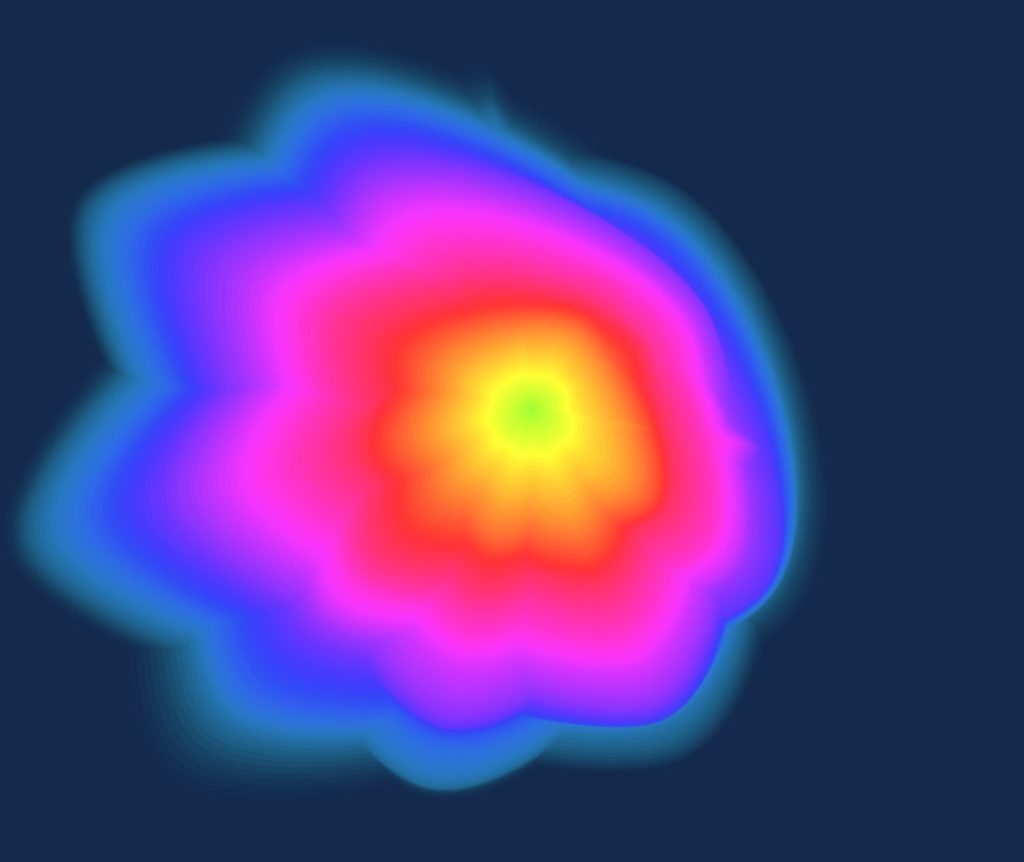